In Ruby, there are two types of variables: class variables and instance variables. These variables serve different purposes and have distinct scopes within the Ruby programming language. Let’s explore the differences between them:
Class Variables
Class variables are prefixed with
@@
and are declared within the class scope.
- Scope: Class variables are shared among all instances of a class, including any subclasses.
- Usage: Class variables are typically used to store data that is shared among multiple objects of the same class.
- Access: Class variables can be accessed and modified by any instance of the class or its subclasses.
Instance Variables
Instance variables are prefixed with
@
and are declared within the instance methods of a class.
- Scope: Each instance of a class has its own set of instance variables, which are unique to that instance.
- Usage: Instance variables are used to store data that is specific to each object (instance) of a class.
- Access: Instance variables are accessible only within the scope of the instance methods of a class.
Class variables are shared among all instances of a class, whereas instance variables are unique to each instance of a class. Class variables are useful when you want to store data that needs to be accessed and modified by all instances, while instance variables are used to hold data specific to each individual instance.
It’s important to note that both class variables and instance variables can be used to maintain state and store data within Ruby classes, but their usage and scope differ. Understanding these differences is crucial for effective and efficient programming in Ruby.
Class variable and instance variable in Ruby example
class Car @@total_cars = 0 # Class variable def initialize(name) @name = name # Instance variable @@total_cars += 1 end def drive puts "#{@name} is driving." end def self.total_cars puts "Total cars created: #{@@total_cars}" end end # Creating instances of the Car class car1 = Car.new("BMW") car2 = Car.new("Mercedes") car3 = Car.new("Audi") car1.drive # Output: BMW is driving. Car.total_cars # Output: Total cars created: 3
In the above example, we have a Car
class that has a class variable @@total_cars
and an instance variable @name
. Here’s how they are used:
- The class variable
@@total_cars
is initialized to 0. In theinitialize
method, whenever a new instance of theCar
class is created, the@@total_cars
is incremented by 1. This keeps track of the total number of cars created. - The instance variable
@name
is assigned with the name passed as an argument to theinitialize
method for each instance of theCar
class. - The
drive
method uses the instance variable@name
to display the name of the car that is currently driving. - The
total_cars
a class method is defined usingself
. It allows us to access the class variable@@total_cars
and display the total number of cars created.
By executing the code, we create three instances of the Car
class: car1
, car2
, and car3
. Each instance has its own @name
instance variable specific to the car’s name. The drive
method is then called on, displaying “BMW is driving.” Finally, the total_cars
class method is invoked, which outputs “Total cars created: 3” since we created three instances of the Car
class.
This example demonstrates how class variables and instance variables are used to store and access data within Ruby classes.
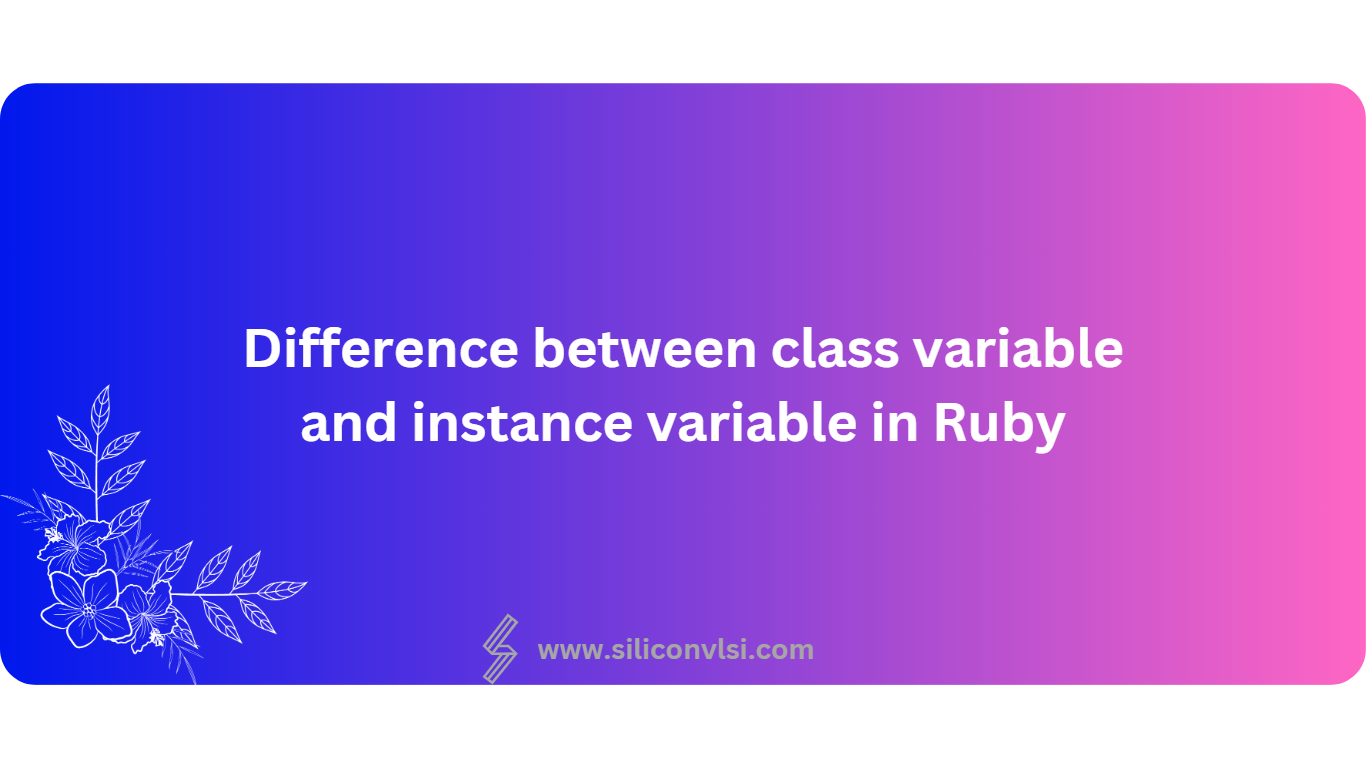