Last-In-First-Out Buffer (LIFO Buffer) – Simplified Explanation
In low-level programming, we deal with data stored in small memory units called “registers.” These registers are closely connected to the processor’s main calculating unit (ALU). They store data temporarily and are essential for the processor’s operations. However, registers are limited in number, and using them efficiently is crucial. Processors need to perform many tasks, and managing these limited registers is a challenge. To address this, registers hold data specific to the current task, rather than holding data from all tasks. Inactive task data is stored in a special memory area called a “stack.”
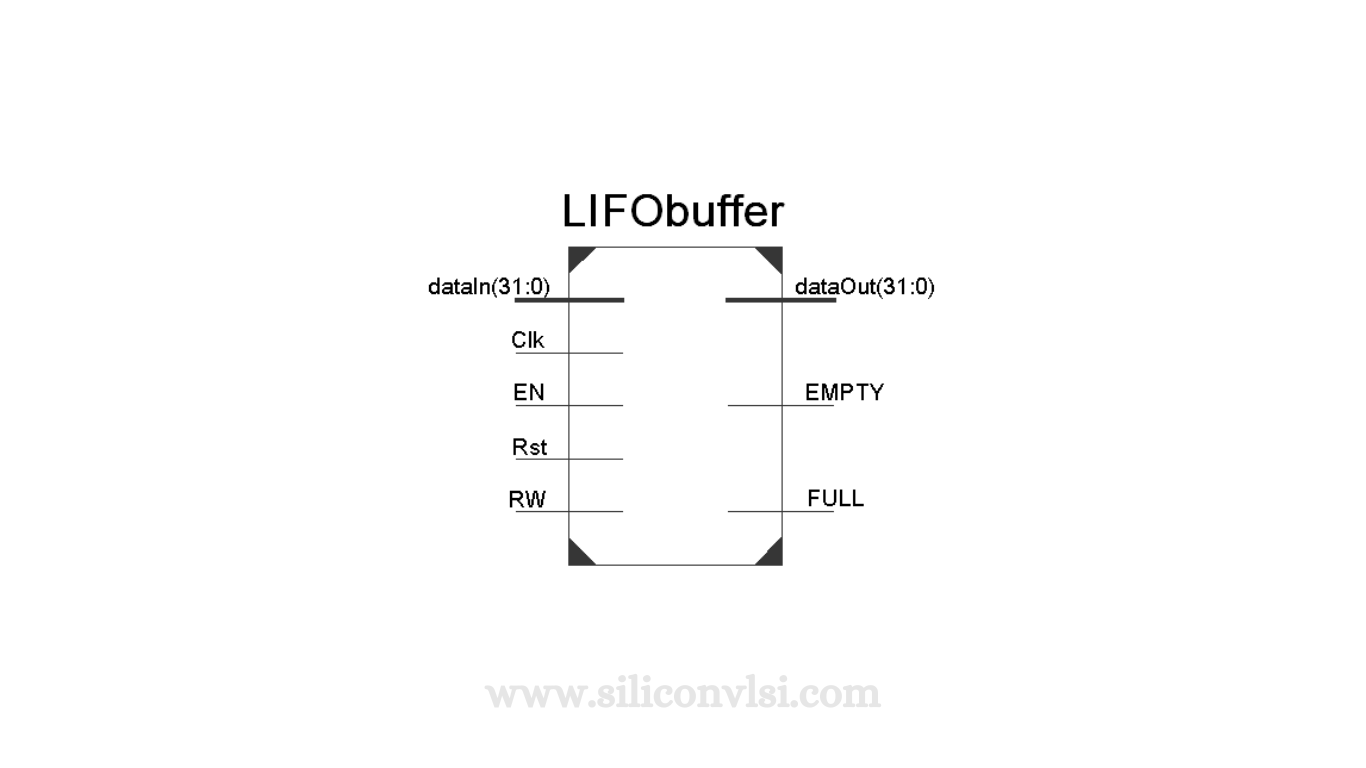
A stack is like a reserved storage area in memory. Processors use it to store data not currently needed but necessary for future steps. Stacks are particularly helpful when using “subroutine” functions. These functions use specific registers, and if those registers are used elsewhere, they can’t be erased. After a subroutine finishes, the original values must be restored. When a processor switches between tasks in a multitasking environment, it needs to store the state of inactive tasks to switch back to them later. This is where the stack comes in handy.
Last In First Out (LIFO) Buffer – Stacks Stacks work in a specific way called “Last In First Out” or LIFO. Imagine a stack of plates where you put new plates on top. When you need a plate, you take it from the top. Similarly, in a computer’s stack, the last piece of data you put in is the first one you take out.
Push and Pop Operations
Push Operation: This is like putting a plate on top of the stack. In computer terms, it means adding data to the stack. When you push, you first reduce the stack’s “pointer” (like counting the number of plates), and then you put the data on top.
Pop Operation: This is like taking a plate from the top of the stack. In the computer, this means removing data from the stack. When you pop, you first take out the top data, then you increase the stack’s “pointer.”
Verilog Module – LIFO Buffer
Think of this like a special machine that works with a stack. It can hold sixteen sets of data, each with 32 bits. It has inputs for putting data in (dataIn), clock (Clk) to control its actions, and signals to tell it when to do certain things (EN, Rst, RW). It also has outputs for getting data out (dataOut), and signals that tell you if it’s full (FULL) or empty (EMPTY).
Verilog Code – LIFO Buffer
This is like telling the machine how to work. It says what happens when you put data in, take data out, and other actions. It’s written in a special language that the machine understands.
module LIFObuffer( dataIn,
dataOut,
RW,
EN,
Rst,
EMPTY,
FULL,
Clk
);
input [3:0] dataIn;
input RW,
EN,
Rst,
Clk;
output reg EMPTY,
FULL;
output reg [3:0] dataOut;
reg [3:0] stack_mem[0:3];
reg [2:0] SP;
integer i;
always @ (posedge Clk)
begin
if (EN==0);
else begin
if (Rst==1) begin
SP = 3’d4;
EMPTY = SP[2];
dataOut = 4’h0;
for (i=0;i<4;i=i+1) begin
stack_mem[i]= 0;
end
end
else if (Rst==0) begin
FULL = SP? 0:1;
EMPTY = SP[2];
dataOut = 4’hx;
if (FULL == 1’b0 && RW == 1’b0) begin
SP = SP-1’b1;
FULL = SP? 0:1;
EMPTY = SP[2];
stack_mem[SP] = dataIn;
end
else if (EMPTY == 1’b0 && RW == 1’b1) begin
dataOut = stack_mem[SP];
stack_mem[SP] = 0;
SP = SP+1;
FULL = SP? 0:1;
EMPTY = SP[2];
end
else;
end
else;
end
end
endmodule
Verilog Test Bench – LIFO Buffer
This is like testing the machine to make sure it works correctly. You tell the machine what to do, like putting data in and taking it out, and see if it does the right thing.
`timescale 1ns / 1ps
module LIFObuffer_tb;
// Inputs
reg [3:0] dataIn;
reg RW;
reg EN;
reg Rst;
reg Clk;
// Outputs
wire [3:0] dataOut;
wire EMPTY;
wire FULL;
// Instantiate the Unit Under Test (UUT)
LIFObuffer uut (
.dataIn(dataIn),
.dataOut(dataOut),
.RW(RW),
.EN(EN),
.Rst(Rst),
.EMPTY(EMPTY),
.FULL(FULL),
.Clk(Clk)
);
initial begin
// Initialize Inputs
dataIn = 4’h0;
RW = 1’b0;
EN = 1’b0;
Rst = 1’b1;
Clk = 1’b0;
// Wait 100 ns for global reset to finish
#100;
// Add stimulus here
EN = 1’b1;
Rst = 1’b1;
#40;
Rst = 1’b0;
RW = 1’b0;
dataIn = 4’h0;
#20;
dataIn = 4’h2;
#20;
dataIn = 4’h4;
#20;
dataIn = 4’h6;
#20;
RW = 1’b1;
end
always #10 Clk = ~Clk;
endmodule
Timing Diagram – LIFO Buffer
This is like a picture that shows when things happen. It helps you understand the order of actions in the machine.
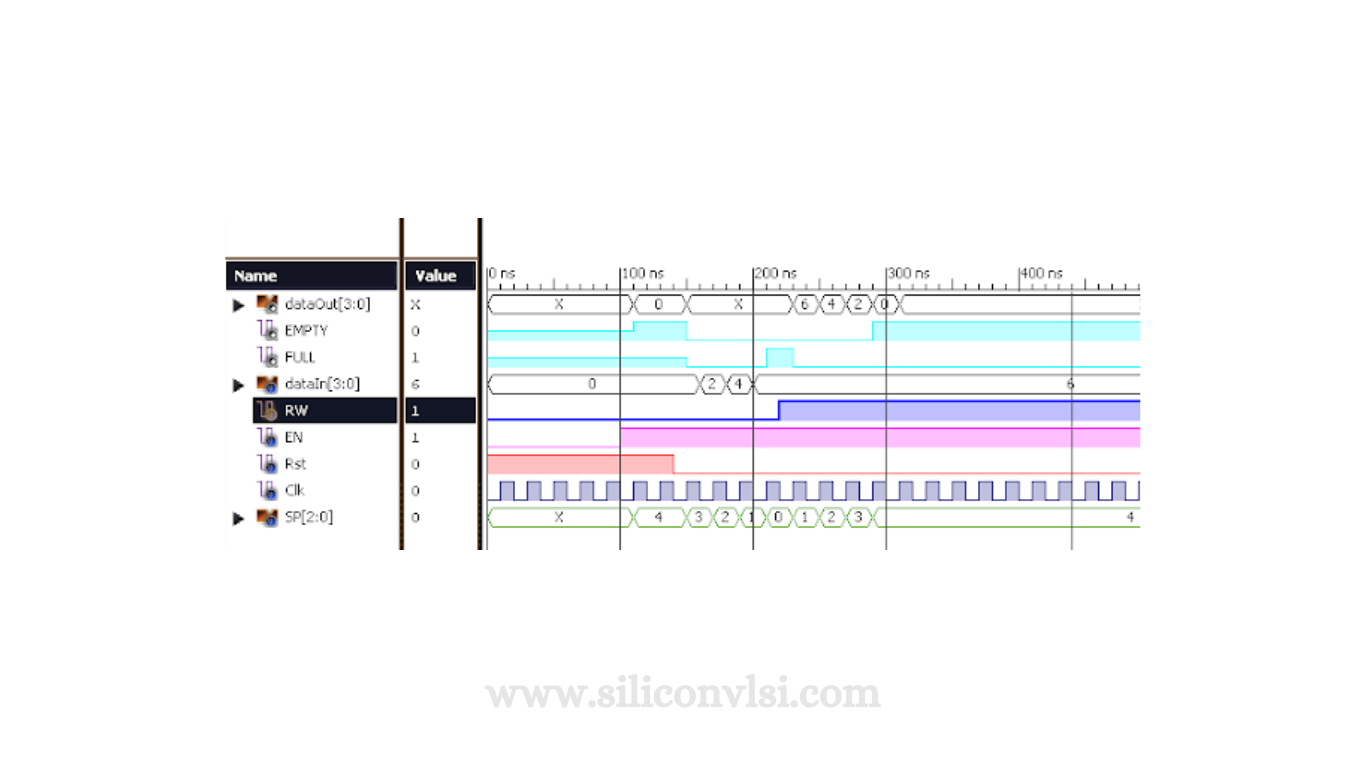
Overall, a Last-In-First-Out Buffer, or LIFO Buffer, is a useful tool for managing data in low-level programming. It helps store and retrieve data efficiently using a stack-like approach