100+ Sasken Interview Questions & Answers – Comprehensive Guide
Prepare for Sasken interviews with this collection of 100+ questions and answers. Get insights, tips, and strategies to excel and land your dream job at Sasken Technologies.
General Programming Concepts
- What is meant by Traversing?
- What is meant by Stack Frames?
- What is a Dangling Pointer?
- What is the Application of Union?
- What is the Critical Section of Code?
- What is meant by Volatile in C?
- What is the Significant of Virtual Memory?
- What are the Types of Data Structures?
- What are the Types of Sorting?
- What is memcpy() and Explain the Syntax?
- What is Sorting and Searching?
Data Structures
- What is a Linked List?
- Difference between Array and Linked List?
- Difference between Linked List and Structure?
- How to Create and Search a Tree?
- How to Find the Number of Nodes in a Tree?
- Write a Program to Add a Node in the Middle in a Single Linked List.
- Write a Program to Swap the Data in a Linked List.
- Write a Program for Binary Search Tree.
- Write a Program for Deleting an Element in a Binary Search Tree.
C and C++ Programming
- Difference between C and Assembly?
- Difference between C and C++?
- Difference between C and Embedded C?
- What is Function Overloading?
- What is Function Overriding?
- What is a Function Pointer?
- Write the Declaration for a Function Pointer That Takes a Char Pointer as Argument.
- What is a Virtual Destructor?
- What printf() Returns and Why Do We Use
&
Operator in Scanf? - Write a Program for String Concatenation.
Operating System and Memory Management
- What is a Process?
- What is a Processor?
- What are the Major Fields in PCB?
- What Are the Scheduling Algorithms?
- Explain the Life of Variables in a Program.
- Explain the Memory Layout of a C Program.
- What Happens to ISR When Interrupt Occurs?
- How to Avoid Deadlock?
- What is a Deadlock?
- How to Avoid Fragmentation?
- What is Meant by Fragmentation?
- What Are the Types of Fragmentation?
- What is Paging and Explain It?
- What is Shared Memory?
- How to Create a Process?
Concurrency and Inter-Process Communication (IPC)
- What is Mutex?
- Difference between Mutex and Semaphore?
- What is a Semaphore?
- What is Inter-Process Communication?
- Name Some IPC Mechanisms.
- What is Race Around Condition?
- What is a Critical Section of Code?
Networking Concepts
- What is the Difference between TCP and UDP?
- What is CAN Protocol?
- What is a Connection-Oriented and Connectionless Mechanism?
Bitwise and Binary Operations
- Write a Program for the Power of 2 (or) Not.
- Write a Program to Print the Status of a Particular Bit in a Number.
- Write a Program to Find Even (or) Odd Numbers Using a Bitwise Operator.
Algorithms and Logical Programs
- Which is the Best Searching and Sorting Algorithm?
- Write a Program for Bubble Sorting.
- Write a Program to Check Whether an Expression is Well-Formed (or) Not.
- Write a Program to Find Out the Missing Elements in an Array.
- Write a Program That Creates an Array in a Function and Returns It to the Main Function.
- How to Create and Search a Tree?
Miscellaneous
- Explain Pre-Processor Stages.
- How Does the OS Know When the Time Slice is Over?
- Where is Memory Allocated to Formal Arguments?
- Where Are Function Pointers Used?
- What is a Timer?
- What is Time Slice?
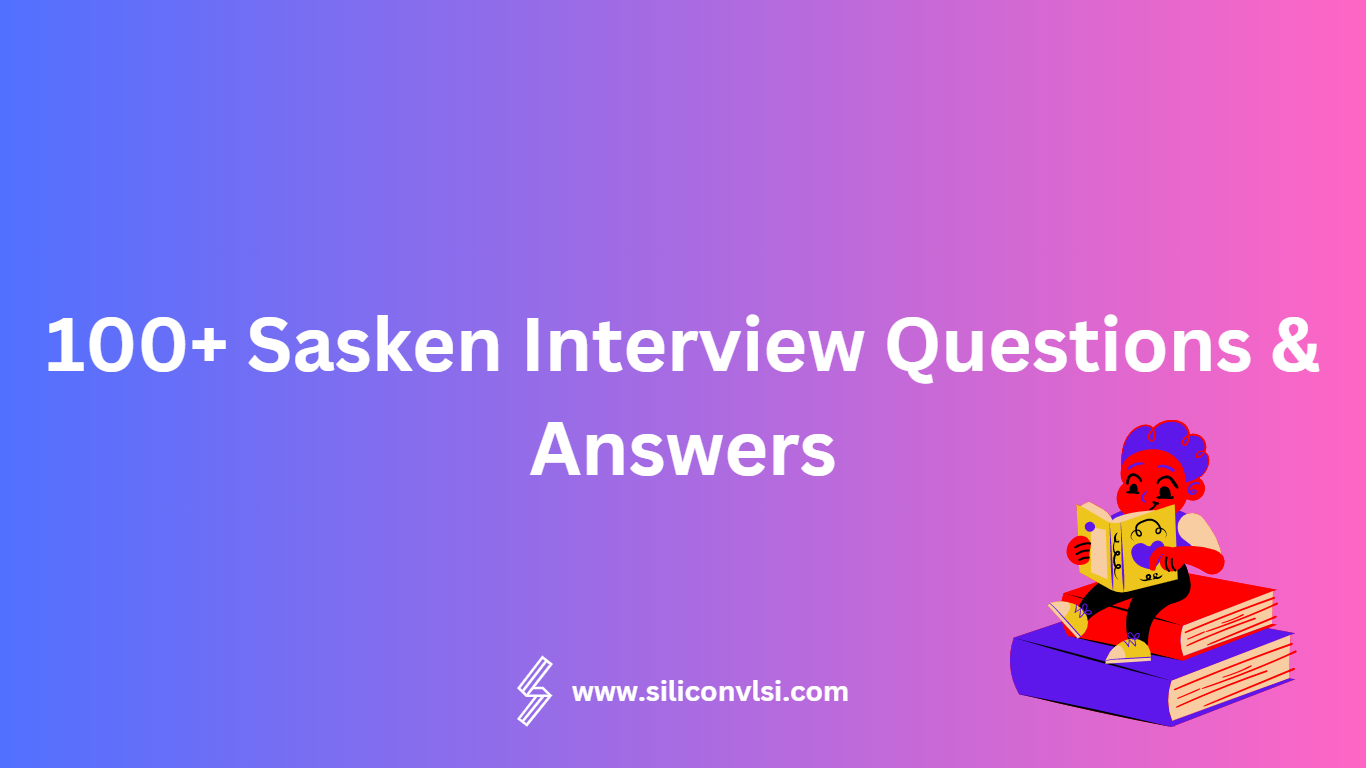