In C#, variable arguments, also known as var args or variable-length parameter lists, provide a way to define methods that can accept a varying number of arguments of different types. This feature allows flexibility in method invocation and is particularly useful when the number of arguments or their types is not known in advance.
C# supports variable arguments through the params
keyword. Here’s an example that demonstrates the usage of variable arguments:
using System; public class Program { public static void Main() { PrintValues("John", 25); PrintValues("Alice", 30, "Manager"); PrintValues("Bob", 35, "Developer", 50000); } public static void PrintValues (string name, params object[] values) { Console.WriteLine("Name: " + name); Console.WriteLine("Additional Values:"); foreach (object value in values) { Console.WriteLine(value); } Console.WriteLine(); } }
Output:
Name: John Additional Values: Name: Alice Additional Values: Manager Name: Bob Additional Values: Developer 50000
In the above example, the PrintValues
method takes a string
parameter, followed by a params
parameter values
of type object[]
. This allows the method to accept any number of additional arguments of different types.
In the Main
method, we invoke the PrintValues
method with different sets of arguments. The first invocation passes only the name
argument, while the subsequent invocations include additional arguments.
Inside the PrintValues
method, we first print the provided name
. Then, using a foreach
loop, we iterate over the values
array and print each value on a new line. The object
type is used to handle arguments of different types.
By using the params
keyword, we can conveniently handle variable arguments in C# methods. This feature simplifies the code and allows for greater flexibility in method invocation, making it easier to work with methods that can accept a variable number of arguments.
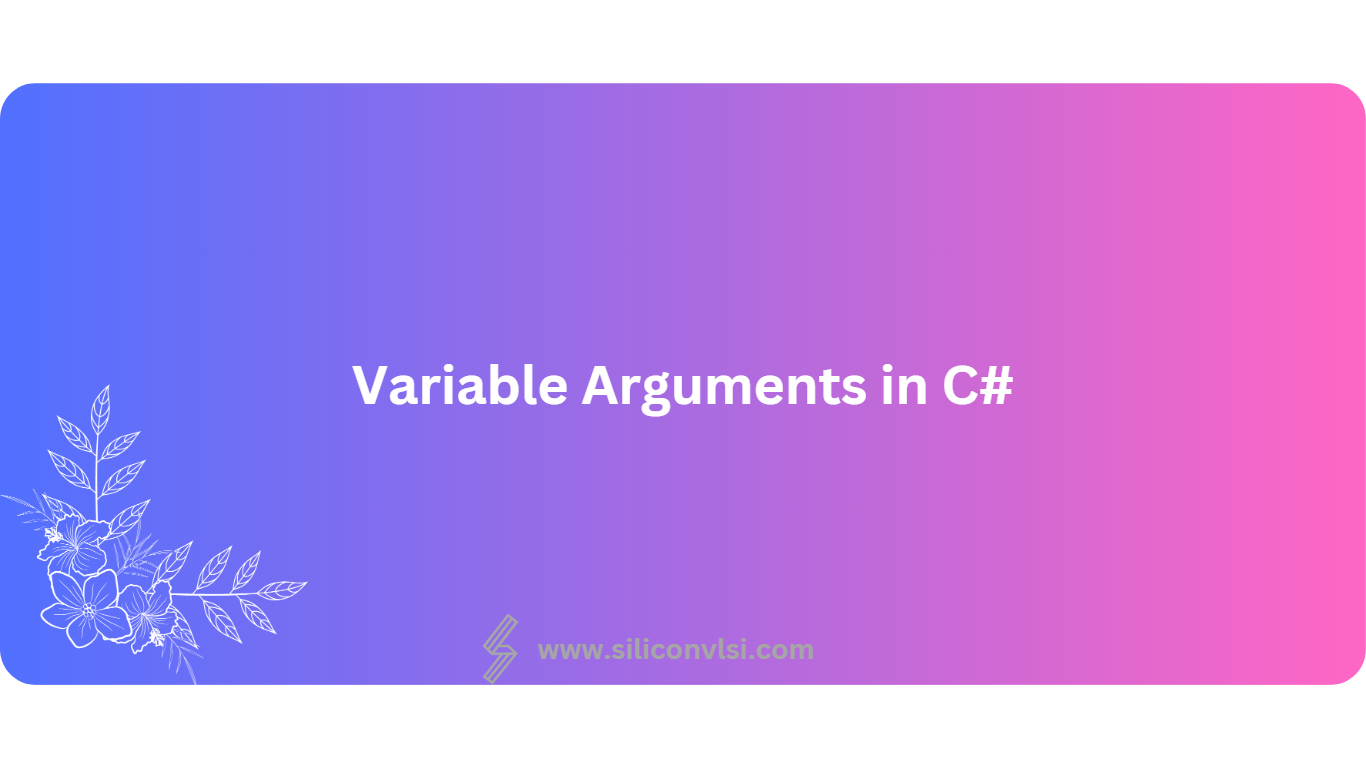