In Verilog, initial and always statements play a crucial role in modeling sequential logic, which is essential for designing digital systems that respond to clock signals. Let’s explore the differences and applications of these statements:
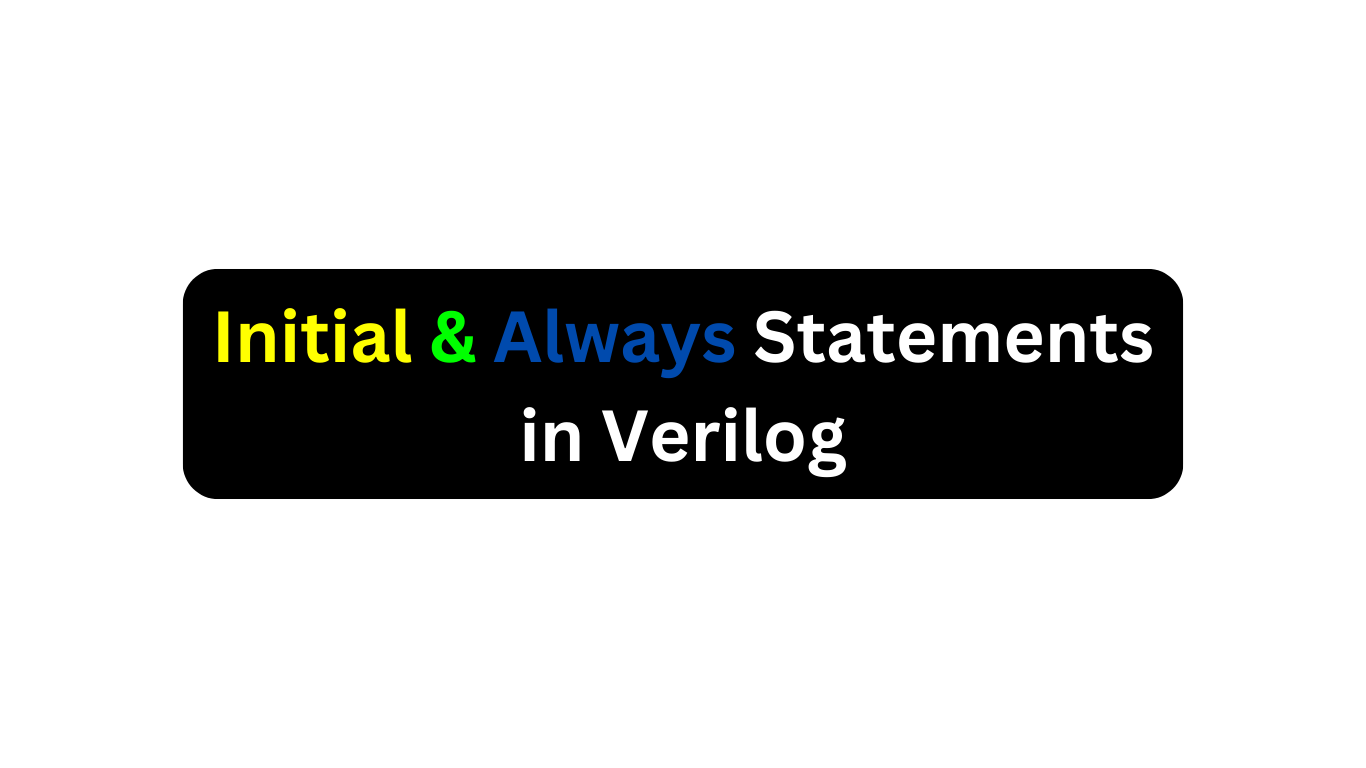
Initial Statements
An initial statement is used to describe a block of code that executes only once at time zero. It is primarily utilized for simulation and verification purposes and is not synthesized into hardware. The basic structure of an initial statement is as follows:
initial begin sequential-statements end
Here, sequential-statements
represent the code to be executed. Initial statements are often used to set up initial conditions, perform simulation-specific tasks, or initialize variables before the simulation begins.
Always Statements: In contrast, an always statement is employed for modeling sequential logic that responds to clock signals or specific events. The fundamental structure of an always statement is as follows:
always @(sensitivity-list) begin sequential-statements end
How Always Statements Different from Initial Statements
Execution Behavior: Always blocks execute over and over again, continuously monitoring the specified conditions. They respond to changes in signals listed in the sensitivity-list
. In contrast, initial blocks execute only once at time zero, regardless of signal changes.
Sensitivity List: The sensitivity-list
in an always statement contains signals that trigger the execution of the block when they change. For example, if the sensitivity list is @(A, B, C)
, the block executes whenever A, B, or C changes. In Verilog 2001 and later versions, a *
can be used in the sensitivity list to execute the block whenever any signal changes.
Synthesis: Always blocks can be synthesized into hardware circuits, making them crucial for designing sequential logic that responds to clock edges. Initial blocks, on the other hand, are not synthesized and are used primarily for simulation and testing.
Data Type: Variables on the left-hand side within an always block should be defined as the reg
data type. Other data types, including wire
, are not allowed.
Concurrent vs. Sequential: Statements outside of an always block, denoted by the assignment operator =
, are concurrent and can execute in any order. Inside an always block, they become sequential statements, executed in the order they are written. This sequential behavior is essential for modeling the step-by-step operations of sequential logic.
In summary, initial statements are used for simulation and one-time setup tasks, while always statements are crucial for modeling synchronous sequential logic that responds to clock signals and events. They enable designers to describe the behavior of digital systems that change over time, making them a fundamental part of Verilog-based digital design.
What is the primary difference between combinatorial logic and synchronous sequential logic in Verilog?
Combinational logic constantly reacts to input changes, while synchronous sequential logic responds to changes dependent on the clock. In synchronous sequential logic, input changes may be ignored, and output or state changes occur only at valid clock conditions.
What are the two types of procedural assignments in Verilog, and how do they differ in execution?
The two types of procedural assignments in Verilog are initial blocks and always blocks. Initial blocks execute only once at time zero, whereas always blocks loop to execute repeatedly. Both types start executing at time zero. Always blocks wait for specific events (sensitivity-list) to occur, while initial blocks execute all statements without waiting.
What is the purpose of a sensitivity list in an always statement in Verilog?
The sensitivity list in an always statement specifies the signals that the process monitors for changes. The process executes the sequential statements inside the always block whenever any signal in the sensitivity list changes.