Unchecked exceptions in Java are exceptions that you don’t need to explicitly handle or declare. These exceptions are subclasses of RuntimeException
and its subclasses.
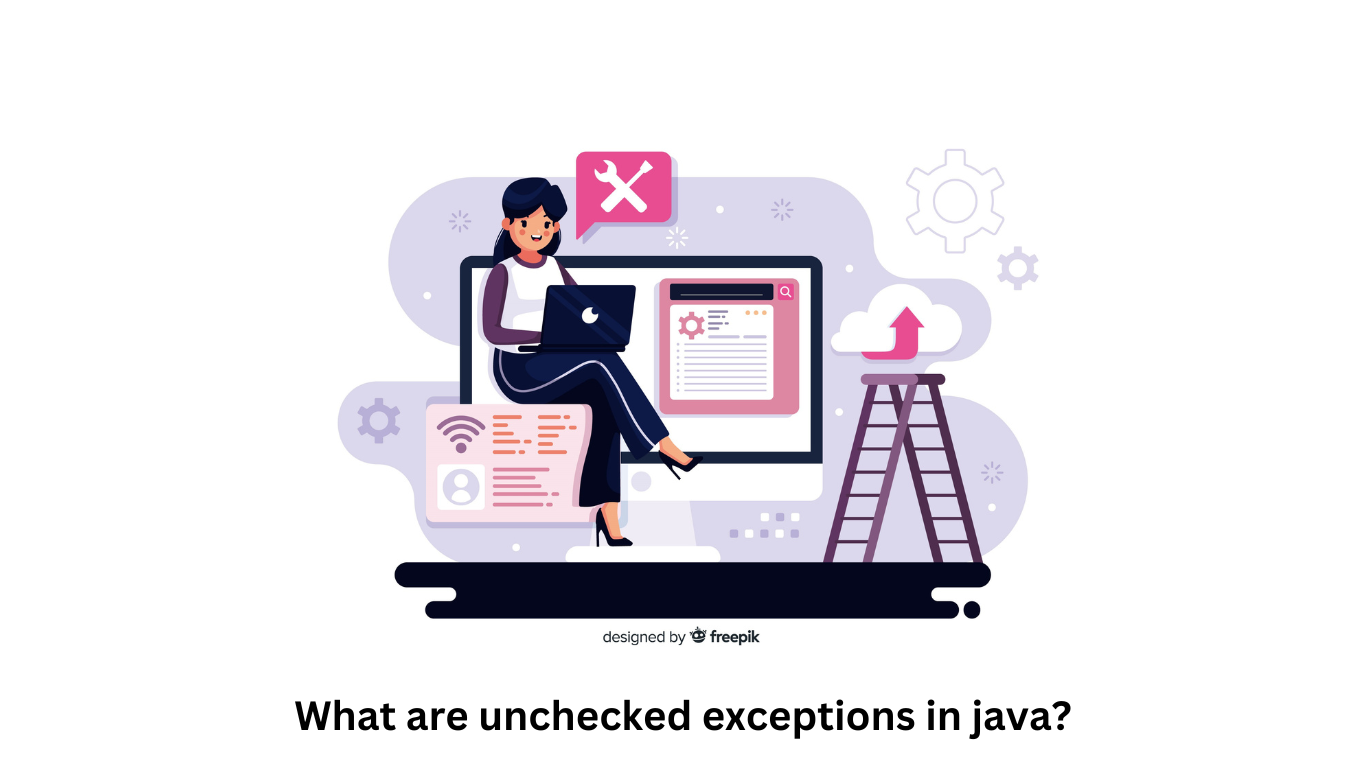
The key characteristics of unchecked exceptions
Examples of unchecked exceptions include:
ArithmeticException: You encounter this when an arithmetic operation produces an error, like division by zero.
ArrayIndexOutOfBoundsException: This happens when you try to access an array element with an invalid index.
ClassCastException: You get this when you cast an object to a class of which it isn’t an instance.
IndexOutOfBoundsException: This is raised when accessing a collection with an invalid index.
NullPointerException: You see this when trying to access a variable that is null.
NumberFormatException: This occurs when you try to convert a string to a numeric type, but the string isn’t properly formatted.
StringIndexOutOfBoundsException: You encounter this when trying to access a character in a string with an invalid index.
UnsupportedOperationException: This is thrown when you try to perform an operation that isn’t supported by the object.
By understanding these exceptions, we can write more robust and error-free code, making our applications more reliable.