What is Inheritance in Java?
Inheritance in Java refers to the technique of adding new functionality to existing classes by adding new members. In Java, we use inheritance to create new classes based on existing ones. This means a child class can reuse methods and fields from a parent class and also add its own. You can achieve method overriding through inheritance, which helps with runtime polymorphism. Inheritance also supports abstraction by showing only the necessary functionality to the user.
I think inheritance in Java is a way to add new features to existing classes. You can create a new class, called a child class, from an original class, known as the parent class. We use the extends
keyword to do this. Every class in Java extends from the Object
class. When you create new classes using inheritance, it creates an ‘is-a’ relationship.
We use inheritance in Java to reuse code and add new functionality. A class that inherits from another class can use the fields and methods of the parent class. You can also add new fields and methods to the child class. This is important for code reusability, method overriding, and abstraction.
You might find inheritance in Java helpful because it allows one class to inherit the features of another class. The parent class is the one being inherited from, and the child class is the one inheriting. Inheritance helps achieve code reusability, so you don’t have to write the same code again. It also allows method overriding and supports abstraction.
I find inheritance in Java to be a fundamental part of Object-Oriented Programming. It lets you create new classes by extending existing ones. The parent class contains common features, and the child class can add more specific ones. We use the extends
keyword for this purpose. Inheritance makes code reusable, allows method overriding, and supports abstraction.
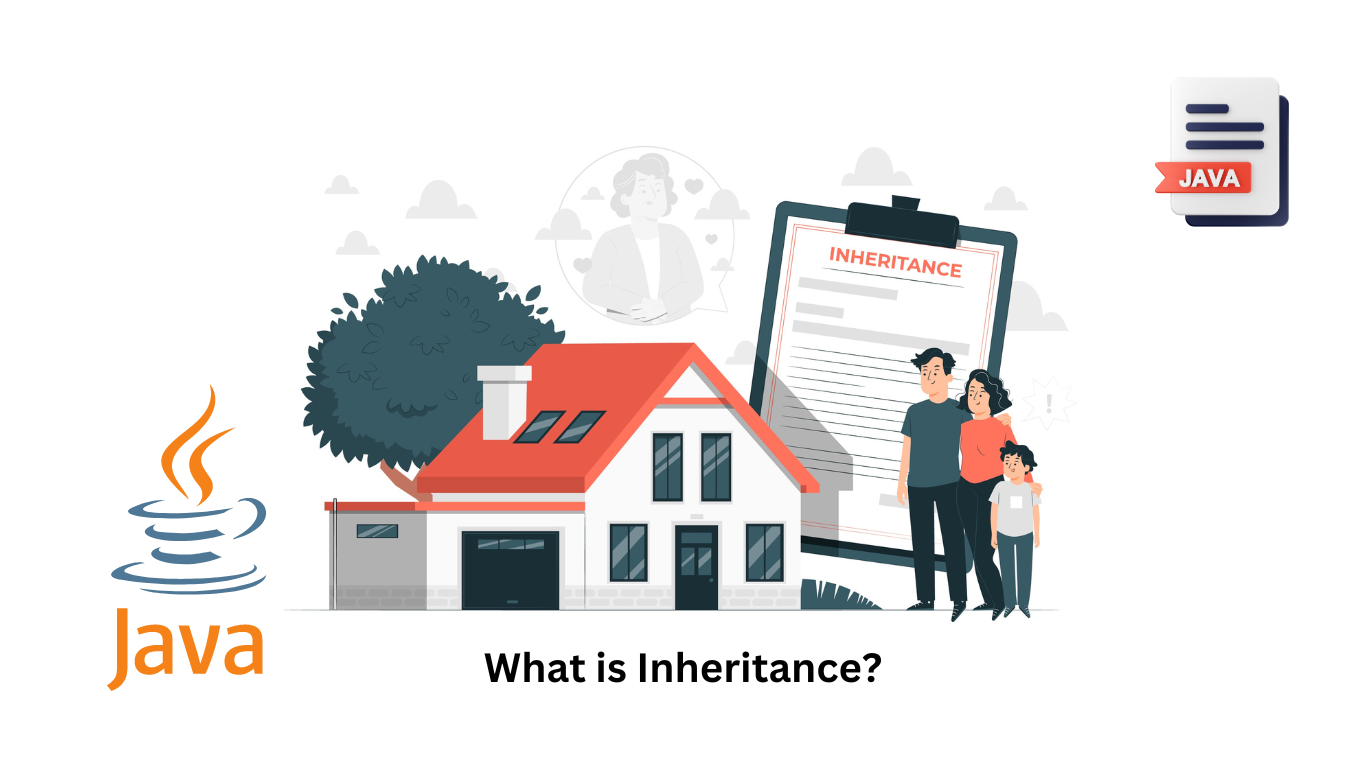
The base class, parent class, or superclass is the original class. The new class that is descended from the parent is referred to as a child class, subclass, or derived class.
To extend a class in Java, use the extends keyword. Every class in Java extends java.lang.since the object class in Java is the superclass for all classes. ‘is-a’ relationships are generated when we create new classes using inheritance.