50+ OOPs Interview Questions and Answers
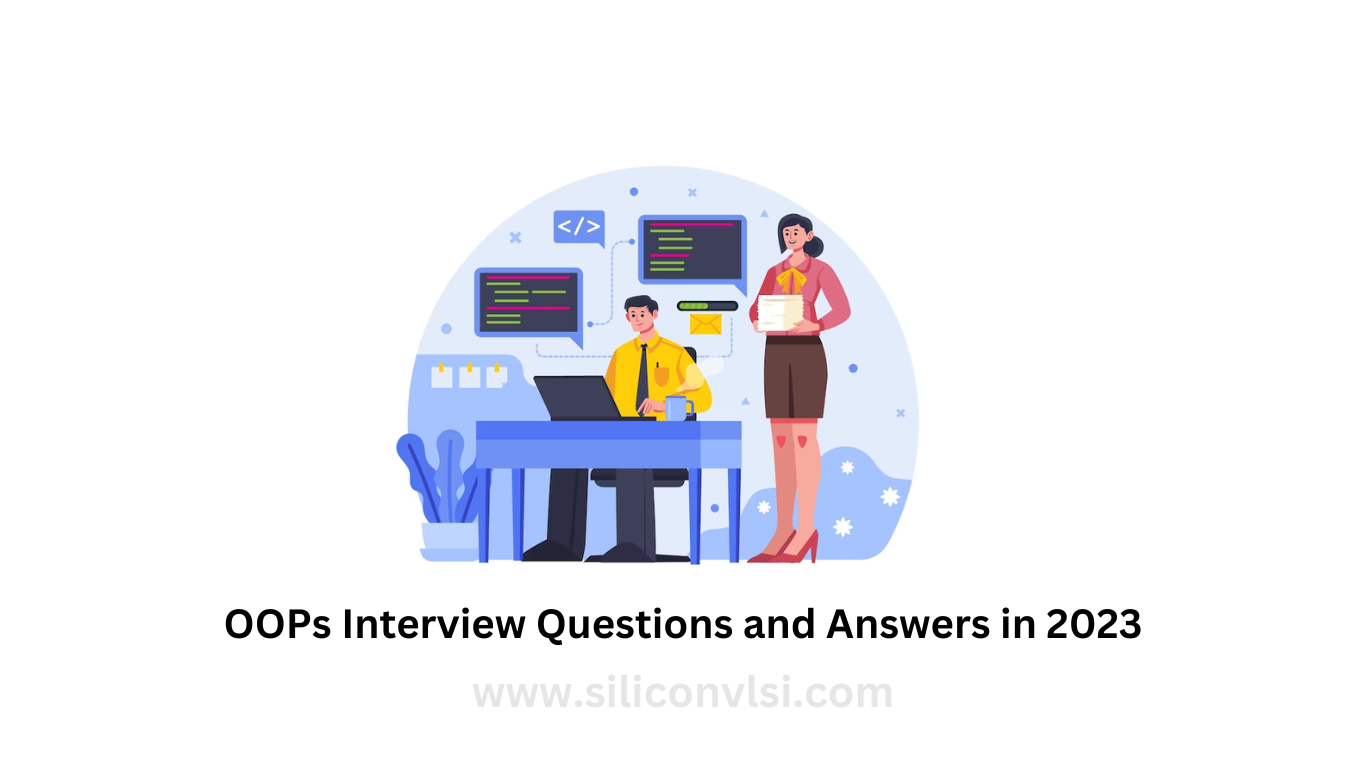
What is OOPS?
OOPS stands for Object-Oriented Programming. It’s a way of writing computer programs where we think about the program as a collection of objects. Each object is like a real-world thing and follows a blueprint called a class.
What is a class?
A class is like a blueprint or template that describes the details of an object. It represents a type of object.
What is an Object?
An object is a specific instance of a class. It has its own characteristics, behavior, and identity.
What is Encapsulation?
Encapsulation is about keeping data hidden within an object. It restricts access to the data to only the members of that class. There are different levels of access like public, protected, and private.
What is Polymorphism?
Polymorphism is when a subclass gives its own behavior or value to something that was already defined in the main class. It means one thing can take multiple forms.
What is Inheritance?
Inheritance is when one class shares the structure and behavior defined in another class. It can be single (one parent class) or multiple (depends on multiple classes).
What is an Abstract Class?
An abstract class can’t be instantiated. It’s like a template for other classes to inherit from. It can have abstract methods that must be defined by its child classes.
What is a Ternary Operator?
The ternary operator is an operator that takes three arguments and behaves based on those arguments. It’s a way to make quick decisions in code.
Use of Finalize Method?
The finalize method helps clean up resources that are not currently in use. It’s called before an object is destroyed.
Different Types of Arguments?
There are two types of arguments: Call by Value (changes made inside the function don’t affect outside) and Call by Reference (changes inside and outside).
What is the Super Keyword?
The super keyword is used to access overridden methods in the parent class. It’s also used to forward calls from a constructor to a constructor in the parent class.
What is Method Overriding?
Method overriding is when a subclass provides its own implementation of a method that’s already defined in the parent class.
Basic Concepts of OOPS
There are four main concepts in OOPS:
Abstraction: Only show the necessary parts of an object to the user.
Encapsulation: Keep the data of an object hidden and controlled.
Inheritance: Share features and behaviors from one class to another.
Polymorphism: Allow objects to take on different forms and behave differently.
What is an Interface?
An interface is a collection of abstract methods. If a class implements an interface, it inherits all the abstract methods of that interface.
What is Exception Handling?
Exception handling deals with events that occur during program execution. Exceptions are errors or unexpected situations. It’s managed using keywords like try, catch, and throw.
What are Tokens?
Tokens are building blocks of code. They can be keywords, identifiers (names for things), constants, string literals, operators, and punctuation characters.
Difference between Overloading and Overriding?
Overloading is when you have multiple methods with the same name but different parameters in the same class. Overriding is when a subclass provides its own version of a method already defined in the parent class.
Difference between Class and Object?
A class is like a blueprint that describes how objects of that class should be. An object is a specific instance created from a class.
What is Abstraction?
Abstraction means showing only the necessary details to the user and hiding the inner workings. It’s about simplifying complexity.
Access Modifiers?
Access modifiers control the scope of methods or variables. There are different types like private, protected, and public.
Sealed Modifiers?
Sealed modifiers prevent methods from being overridden. They’re used to restrict further modification.
Calling Base Method without Instance?
You can call a base method without creating an instance by using the base keyword from a derived class.
Difference between New and Override?
The new modifier uses a new implementation instead of the base class function, while the override modifier overrides the base class function.
What are Manipulators?
Manipulators are functions used with the << and >> operators on objects. They help control how data is displayed or extracted. Examples are endl and setw.
Explain Constructor
A constructor is a method used to set up the initial state of an object when it’s created. It’s automatically called when an object is created. It should have the same name as the class and no return type.
Define Destructor
A destructor is a method that’s automatically called when an object goes out of scope or is destroyed. It’s used to clean up resources used by the object.
What is an Inline Function?
An inline function is a technique where the code of the function is inserted directly where it’s used in the program. It helps save time during execution.
What is a Virtual Function?
A virtual function is a method in a class that can be overridden in its derived class. It’s marked with the keyword virtual.
What is a Friend Function?
A friend function is allowed to access the private, protected, or public data of a class. It’s not affected by access control keywords like private or public.
What is Function Overloading?
Function overloading means having multiple methods with the same name but different parameters. This allows the same function name to do different things based on the input.
What is Operator Overloading?
Operator overloading lets you define what operators like + or – do for objects of a class. It allows you to give special meanings to operators.
Types of Constructors?
There are three types: Default Constructor (no parameters), Parametric Constructor (with parameters), and Copy Constructor (creates a copy of an existing object).
What is Early and Late Binding?
Early binding associates a name with a class during compilation, while late binding associates a name with a class during runtime.
What is the ‘this’ Pointer?
The ‘this’ pointer refers to the current object of a class. It’s used to differentiate between the current object and the global object.
Difference between Structure and Class?
A structure is used for grouping data, while a class can group data and methods. A structure’s default access is public, while a class’s is private.
Default Access Specifier in Class?
The default access specifier in a class is private.
What is a Pure Virtual Function?
A pure virtual function is a function in an abstract class that doesn’t have a defined implementation. It’s marked with = 0.
Operators that cannot be Overloaded?
Operators like ::, ., and .* cannot be overloaded.
Dynamic or Runtime Polymorphism?
Dynamic or runtime polymorphism is when a subclass’s method overrides the parent class’s method. It’s resolved during runtime.
Parameters for Constructors?
Constructors don’t require parameters, but you can have constructors with parameters.
Copy Constructor?
A copy constructor creates a new object as a copy of an existing object.
What does the Keyword ‘Virtual’ Represent?
The virtual keyword is used to declare a method that can be overridden in derived classes.
Can a Static Method use Non-static Members?
No, a static method can’t use non-static members.
Base Class, Subclass, and Superclass?
The base class is the main class that can be inherited. A subclass is a class that inherits from another class. A superclass is the parent class from which another class inherits.
Static and Dynamic Binding?
Static binding is when a name is associated with a class during compilation (early binding). Dynamic binding is when a name is associated with a class during runtime (late binding).
Instances for an Abstract Class?
You can’t create instances of an abstract class.
Keyword for Operator Overloading?
The keyword for operator overloading is operator.
Default Access Specifier in Class Definition?
The default access specifier in a class definition is private.
OOPS Concept for Reuse Mechanism?
The OOPS concept used for reuse is Inheritance.
Concept that Exposes Necessary Information?
The concept that exposes necessary information is Encapsulation